Being able to start one application from another is
one of the significant advantages of Windows. Within Visual Basic you
may want to start another application to load data from Excel, to
display a web page, to edit text files, or to perform some other task
not easily done in Excel itself. Visual Basic provides the functions
shown in Table 1 to run other applications.
Table 1. Visual Basic functions for running other applications
Function | Use to |
---|
AppActivate | Switch focus to a running application |
CreateObject | Start an ActiveX application and get an object reference to that application |
GetObject | Get a running ActiveX application and get an object reference to that application |
SendKeys | Send keystrokes to a running Windows application |
Shell | Start an application using its file (.exe) name |
CreateObject and GetObject work only with Windows applications that have support for ActiveX automation
built in to them. Most Microsoft products and many other Windows
products support that type of automation, which is sometimes also called
OLE automation
.
ActiveX or OLE
automation allows you to use the internal objects, properties, and
methods of the application in the same way that you control Excel from
Visual Basic. For example, the following code starts Microsoft Word from
Excel, creates a new document, inserts some text, and saves the file:
Sub UseWord( )
Dim word As Object, doc As Object
Set word = CreateObject("Word.Application")
' Show Word (otherwise it's invisible).
word.Visible = True
' Create document.
Set doc = word.Documents.Add
' Insert some text
doc.Range.InsertAfter "Some text to insert."
' Save the file
doc.SaveAs ThisWorkbook.Path & "\StartWord.doc"
End Sub
You can use GetObject
to get a running instance of an application. For example, the following
code uses a running instance of Word to open the document created by
the preceding code:
Sub GetWord( )
Dim word As Object, doc As Object
' Get a running instance of Word.
Set word = GetObject(, "Word.Application")
' Open a document.
Set doc = word.documents.Open(ThisWorkbook.Path & "\StartWord.doc")
word.Visible = True
End Sub
In the preceding code, GetObject fails if Word is not already running. You can use GetObject with a filename to start the application associated with the file if it is not already running, as shown here:
Sub GetDocument( )
Dim word As Object, doc As Object
' Get the demo document whether or not is it open.
Set doc = GetObject(ThisWorkbook.Path & "\StartWord.doc")
' Show the document.
doc.Application.Visible = True
End Sub
In this case, GetObject
starts Word if it is not already running and loads the document. If
Word is running or if the document is already open, the code just
returns a reference to that object without starting a new instance.
Both CreateObject and GetObject do something called late binding
. Late binding means that the type of the object is not checked until the application is running. It's often better to use early binding
, since that allows Visual Basic to check that types match when the code is compiled, which helps correct type-mismatch errors.
Early binding requires that
you add a reference to the library for the application you want to use
from Excel. To add a reference to an application in Visual Basic:
Select Tools → References. Visual Basic displays the References dialog box, shown in Figure 1.
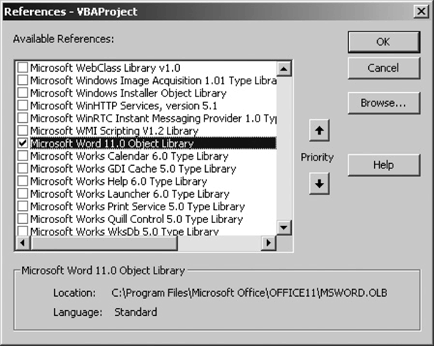
Scroll
down the list of applications installed on your system and select the
ones you want by clicking the box next to the application's name.
There may be quite a
few libraries installed on your computer, and sometimes it is difficult
to find the one you want. Microsoft groups most of its libraries under
the company name so they sort together, and most other companies do the
same.
If
you distribute your Visual Basic code to others, any applications you
use must also be installed on their computer, otherwise the code will
fail. |
|
Once you have added a
reference to another application, you can use objects from that
application in the same way that you use Excel objects. For example, the
following code creates a new document in Word and inserts some text:
' Requires reference to Microsoft Word object library.
Sub EarlyBinding( )
Dim doc As New Word.Document
doc.Range.InsertAfter "This is early-bound."
doc.Application.Visible = True
End Sub
One of the key advantages of using explicit types, such as Word.Document,
is that it enables Visual Basic's Auto Complete features to help you
navigate through the various objects, properties, and methods that an
application provides. Figure 2 shows the Auto Complete feature in action for the Word objects.
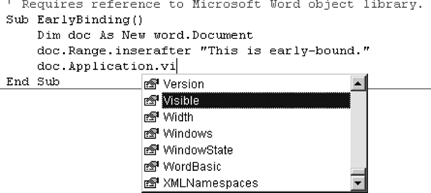
Table 2
lists some common applications that provide ActiveX objects you can use
from Visual Basic. Be aware that most of these applications provide a
very complex set of objects that may be organized differently from
Excel's objects. Using the objects from other applications often
requires a good deal of research and learning.
Table 2. Some applications that you can automate from Visual Basic
Application name | Library name (for References dialog) | Programmatic IDs (for CreateObject/GetObject) |
---|
Microsoft Word | Microsoft Word 11.0 Object Library | Word.Application
Word.Document |
Microsoft PowerPoint | Microsoft PowerPoint 11.0 Object Library | PowerPoint.Application
PowerPoint.Show
PowerPoint.Slide |
Microsoft Access | Microsoft Access 11.0 Object Library | Access.Application
Access.Workgroup
Access.Project |
Microsoft Excel | Microsoft Excel 11.0 Object Library | Excel.Application
Excel.Sheet
Excel.Chart |
Microsoft Graph | Microsoft Graph 11.0 Object Library | MSGraph.Application
MSGraph.Chart |
Microsoft Outlook | Microsoft Outlook 11.0 Object Library | Outlook.Application |
Microsoft Internet Explorer | Microsoft Internet Controls | InternetExplorer.Application |
Not all applications support ActiveX, however. Some of the simple (and common) applications can be started only with the Shell function. Shell runs the application using its filename and returns a nonzero number if the application started successfully. You can combine Shell with SendKeys to automate applications in a simple way:
Sub StartNotepad( )
Dim id As Integer
id = Shell("notepad.exe", vbNormalFocus)
' If id is not zero, then Shell worked. This is some text to insert
If id Then
SendKeys "This is some text to insert", True
SendKeys "^s", True
SendKeys ThisWorkbook.Path & "\StartNote.txt", True
SendKeys "~", True
End If
End Sub
The preceding code starts
Notepad, inserts some text, and saves the file. You can send keystrokes
to only the application that currently has focus in Windows. If the
user changes the focus while your code is running, the keystrokes may go
to the wrong application. This makes debugging the preceding code very
difficult. If you try to step through the code, the keystrokes are sent
to the Code window, rather than to Notepad!
You can switch the focus to another application using the AppActivate function. AppActivate
uses the text displayed in the window's titlebar to select the window
to grant focus. If you run the preceding code, the Notepad window will
contain the text "StartNote.txt — Notepad", so the following code will
switch focus to that window to make some changes:
Sub GetNotepad( )
Dim id As Integer
On Error Resume Next
AppActivate "StartNote.txt - Notepad", True
If Err Then MsgBox ("StartNote.txt is not open."): Exit Sub
SendKeys "{end}", True
SendKeys ". Some more text to insert.", True
SendKeys "^s", True
End Sub
You can also use the ID returned by the Shell function with AppActivate to activate a running application. SendKeys uses the predefined codes
listed in Table 3 to send special keys, such as Enter or Page Up, to an application.
Table 3. SendKeys codes
Key | Code | Key | Code |
---|
Shift | + | Ctrl | ^ |
Alt | % | Backspace | {BACKSPACE}, {BS}, or {BKSP} |
Break | {BREAK} | Caps Lock | {CAPSLOCK} |
Del or Delete | {DELETE} or {DEL} | Down Arrow | {DOWN} |
End | {END} | Enter | {ENTER} or ~ |
Esc | {ESC} | Help | {HELP} |
Home | {HOME} | Ins or Insert | {INSERT} or {INS} |
Left Arrow | {LEFT} | Num Lock | {NUMLOCK} |
Page Down | {PGDN} | Page Up | {PGUP} |
Print Screen | {PRTSC} | Right Arrow | {RIGHT} |
Scroll Lock | {SCROLLLOCK} | Tab | {TAB} |
Up Arrow | {UP} | F1-F16 | {F1}-{F16} |
I should point out here that Excel provides methods for running two of the most commonly used applications. Use the FollowHyperlink method to display a web page in the default browser or to create a new email message. Use the SendMail method to send a workbook as an attachment. The following code demonstrates these different approaches:
Sub BrowserAndMail( )
' Starts browser and displays page.
ThisWorkbook.FollowHyperlink "http://www.mstrainingkits.com"
' Creates a new, blank mail message
ThisWorkbook.FollowHyperlink "mailto:[email protected]"
' Sends the workbook as an attachment without displaying message.
ThisWorkbook.SendMail "[email protected]"
End Sub